Adding Multiselection to TFileOpenDialog
Date: 7 February
1997
Author: Brice VIDAL
Platform: 32 bits environment
In memory of: Carcass
Overview
- An advantage of MDI applications
is the ability to open several files from a directory at a time. When using
a TFileOpenDialog, to enable multiselection you must set
the OFN_ALLOWMULTISELECT flag in the TOpenSaveDialog::TData
structure passed to the dialog.
- Then starts the real work: decode the
filenames. The string returned is kind of formatted: if 1 file has
been selected then the full path of the filename is returned, but
if several files have been selected then the path is first, followed by
each file name. Each item is separated by a null terminator
char and the end of the string is delimited by 2 null terminator
characters. You can download the code here, it
is completely documented so do not hesitate.
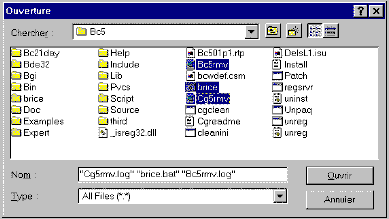
Setting the OFN_ALLOWMULTISELECT flag
- Generally this flag
is set in the SetupWindow function of your MDI class, although you can define
it just before opening the dialog box. All you need is set the flags
of the TOpenSaveDialog::TData struct that will be passed to
the constructor. Have a look at your help file for available flags.
- If you want an explorer
type (common win95 open dialog boxes) dialog box allowing multiselection
here's the first step:
#include <owl/opensave.h>
TOpenSaveDialog::TData FileData;
FileData.Flags = OFN_EXPLORER | OFN_ALLOWMULTISELECT;
Managing the result
Then starts the real work.
- First, we create, call and check the
return value of the dialog box:
#include <dir.h>
#include <cstring.h>
if(TFileOpenDialog(this, FileData).Execute()==ID_OK)
{
- Then we need to know how many files have
been selected. So we get the current working directory with getcwd()
and compare its number of letters to the one of the FileData.FileName
string with strlen() because the strlen function calculates
the number of letters until it reaches a null terminator and then
returns this number without counting the null terminator. So if the directory's
length is the same as the FileData.FileName one it means that several files
have been selected.
char currentDirectory[MAXPATH];
getcwd(curPath, MAXPATH);
int size = strlen(FileData.FileName);
if(strlen(curPath) != size)
OpenFile();
else
{
- The next step is to open each file. For
this we create a string containing the directory. A while loop starts until
the length of what is behind the null terminator is 0. If there
is a file name we collect it and create a string composed of the directory
to which we add the file name and then a call to OpenFile()
does all the work.
char* path =
FileData.FileName;
bool exit = false;
string dir(path);
dir += "\\";
while(!exit)
{
char* file = FileData.FileName
+ size + 1; // skip the null terminator
int fileSize = strlen(file);
if(!fileSize)
exit
= true;
else
{
size
+= (fileSize + 1);
string
openName(file);
openName
= dir + openName;
OpenFile(openName.c_str());
}
}
}
}
Here's an example of an OpenFile() function:
void OpenFile(const char* fileName = 0)
{
if(!fileName)
// the FileData.FileName
contains the file name
That's it, you now have a multifile open dialog
box !
Now if you need more information, jump to the
advanced page.